Introduction
Web Vitals scores can have a huge influence on your search engine rankings and directly impact your user’s experience when visiting your website. Follow our guide to automating Web Vitals checks against your Vercel CI Deployment using GitHub Actions and ensure that you don’t introduce performance issues into your code that could affect your search engine ranking.
Web Vitals and Lighthouse
As we covered previously in our Size Matters: The Value of Performance Optimization post, your Web Vitals scores will directly impact your search engine ranking. We’ve spent a lot of time and effort optimizing our own website to meet the Web Vitals performance requirements and have audited this using the Lighthouse tool.
Lighthouse is an open-source tool used to audit your website performance in a controlled environment. It provides scores for the key Web Vitals including the first contentful paint, speed index, time to interactive, and other criteria. These are combined and weighted to provide your site with a score out of 100 for performance, accessibility, best practices, SEO, and performance as a Progressive Web App (PWA).
Until recently, we have been using a manual process to run the Lighthouse audit against our site, but now we have set up Github Actions to run the audit automatically for us for each pull request that is raised. This means that we can now assess the impact on our Web Vitals score for any change we make to our site.
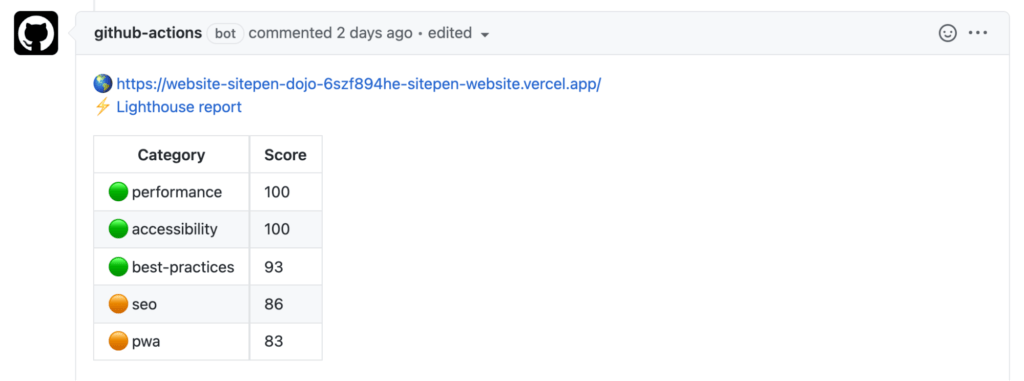
Lighthouse Budgets
Lighthouse allows us to provide a budget file that specifies requirements for each of the Web Vitals measurements such as first-contentful-paint
and cumulative-layout-shift
. You can also specify the maximum sizes for image loads, script loads, and the number of third-party requests your page can make. Further details on the available budget properties are well documented here.
For our website, we have set budgets that ensure we keep a green
score for each of the main areas and limit our JS and IMG sizes for performance. This will ensure that we get the best possible Web Vitals scores and that we do not drop below the levels Lighthouse considers to be top tier.

If the Lighthouse audit fails to meet set budgets, assertion results are returned that describe where the problems occurred with a link to further details of the assertion and what was expected.
This assertion output allows the user to work out what was wrong and provides a link to the Lighthouse report which can provide further information about the audit in general.
The budget file is named budget.json
and is located in the .github/lighthouse/
directory. Below is an example budget file showing the various metrics you can assert against.
[
{
"path": "/*",
"timings": [
{
"metric": "interactive",
"budget": 3000
},
{
"metric": "first-contentful-paint",
"budget": 1800
}
],
"resourceSizes": [
{
"resourceType": "script",
"budget": 100
}
],
"resourceCounts": [
{
"resourceType": "third-party",
"budget": 4
}
]
}
]
Writing the Github Action
GitHub Actions allow you to write a workflow within your repository code that can be triggered by a number of GitHub events such as pushes, pull requests, comments, and others. There are a number of off-the-shelf actions available from the GitHub Marketplace that can be used to call a number of external services and APIs such as vercel
or lighthouse-ci
.
When an action is triggered by a pull-request
event, the outcome of the action can be used to pass or fail the pull request checks and can even be selected as a mandatory check within the branch protection settings. For our GitHub Action, we’re going to create a pull_request_audit.yml
file in the .github/workflows
directory. When you raise a pull request that includes this file, the actions will be picked up and run.
In order to perform a Lighthouse audit on our pull request changes, we needed to:
- Deploy our changes using Vercel
- Run Lighthouse against the Vercel preview URL
- Format the results
- Display the results on the pull request
Let’s take a look at the Marketplace actions we need and how to use them.
Vercel Action
The Vercel Action allows us to trigger a Vercel deployment of our pull request code and return a preview URL which we can use to check the deployment before merging. To use the Vercel action you will need to ensure that you are not using a GitHub integration within your current Vercel setup and that you have linked your project to Vercel via the Vercel CLI in order to get the project_id
and org_id
information as per the following Vercel Project Linking documentation.
You will need to save the Vercel ids as GitHub secrets
within your repository under Settings > Secrets > New repository Secret
. If you are using Vercel as a team you will need to set the Vercel scope
here as well and optionally set it up as a GitHub secret.
We use Vercel to deploy the SitePen website so we have included the Vercel Action in this guide. If you are using GitHub Pages, Netlify, or other platforms, you can remove the vercel-action
code from the workflow and place your own URLs in the lighthouse-action
instead.
Here is the config we are using to trigger a Vercel deployment for our project using a Vercel team scope.
- uses: actions/checkout@v2
- name: Vercel Action
id: vercel_action
uses: amondnet/vercel-action@v20
with:
vercel-token: ${{ secrets.VERCEL_TOKEN }}
github-token: ${{ secrets.GITHUB_TOKEN }}
vercel-org-id: ${{ secrets.ORG_ID}}
vercel-project-id: ${{ secrets.PROJECT_ID}}
scope: ${{ secrets.VERCEL_SCOPE }}
After this has run, the preview-url
is output from the action and can be accessed via steps.vercel_action.outputs.preview-url
in subsequent actions.
Lighthouse CI Action
The Lighthouse CI Action allows you to run a Lighthouse audit against specified URLs. In this case, we wish to use the preview-url
from the Vercel action in order to audit our PR deployment.
In order to ensure we are meeting our Web Vitals targets, we set a budget
path here which will ensure that assertions are run on each URL and if a budget assertion fails, the build will fail.
We set up Lighthouse to perform 3 runs on each of the URLs we pass in. Lighthouse then picks out the median result to assert against. At the moment, we are running audits on our home page and our blog landing page, but you have the option to add more URLs as needed.
After the action is complete, both the Lighthouse score and the assertion results are output and can be accessed via the following:
steps.lighthouse_audit.outputs.manifest
steps.lighthouse_audit.outputs.assertionResults
- name: Audit URLs using Lighthouse
id: lighthouse_audit
uses: treosh/lighthouse-ci-action@v7
with:
urls: |
${{ steps.vercel_action.outputs.preview-url }}
${{ steps.vercel_action.outputs.preview-url }}/blog
budgetPath: '.github/lighthouse/budget.json'
uploadArtifacts: true
temporaryPublicStorage: true
runs: 3
Displaying the Results
To best display the results of the audit, we need to get hold of the Lighthouse audit output and format the results. We can then display these on the pull request using the sticky-pull-request-comment action. This action allows sticky comments to be added to a pull request and later edited.
When calling the sticky comment action you must ensure that you set a header
and use the same name consistently. This will ensure that the correct comment gets updated.
- name: Add comment to PR
id: loading_lighthouse_comment_to_pr
uses: marocchino/sticky-pull-request-comment@v2
with:
GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
number: ${{ github.event.pull_request.number }}
header: lighthouse
message: |
🚦 Running Lighthouse audit...
To format the assertion results, if there are any, you can simply loop through them and build up a string to pass as message
to the comment
action with the correct header
.
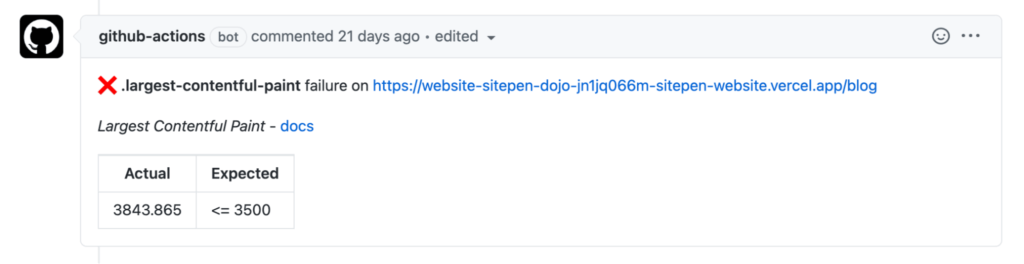
Putting it All Together
The complete finished action can be found in the vercel-lighthouse-action repository along with an example budget.
It will deploy your PR code to Vercel, run three Lighthouse audits against the provided URLs based on the Vercel preview URL, and then format the results before posting them as a comment onto the PR.
Summary
Adding Lighthouse checks to your pull request workflow will ensure that your website meets important Web Vitals standards and keep your SEO scores high. Utilizing a budget can immediately alert your engineers to changes that will have a negative impact on your Web Vitals scores without needing to perform lengthy manual audits.
SitePen can provide assistance on all kinds of website performance including Web Vitals and Lighthouse audits. For more information please get in touch.